Send Post Data While Redirecting With JQuery
Do you want to redirect after form submit in jquery and want to know how to redirect to another page after submitting form data in jquery ? Here is a way to submit data in jquery and redirect to another page.
To send post data while redirecting from one page to another, we should create a dummy form and hidden input field.
Hidden input fields will be the actual data we need to post.
Pass values to this form in textbox and it will be passed from one page to another.
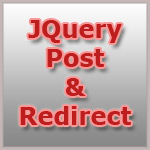
var myRedirect = function(redirectUrl) { var form = $('<form action="' + redirectUrl + '" method="post">' + '<input type="hidden" name="parameter1" value="sample" />' + '<input type="hidden" name="parameter2" value="Sample data 2" />' + '</form>'); $('body').append(form); $(form).submit(); };
Now just assign this variable to the onclick handler of any element. This is a simple way to submit data to another page dynamically when redirecting or moving from one page to another.
Let me know if you know any other way to do this.
Leave a Reply